In this small post we will learn how to show alerts in iOS, alerts are most important things in iOS applications, for example while developing applications you must tell the user that by doing this action your data will be removed you can not undo it or something that you want to inform the user about the action that he is going to make will effect the user flow.
Most of the applications uses alert views to inform the user or take the action or many other use cases of alert views, so now in this post we will see how to create and configure alert views in iOS.
First step create a new project and name it as Alert-Example as shown below image.
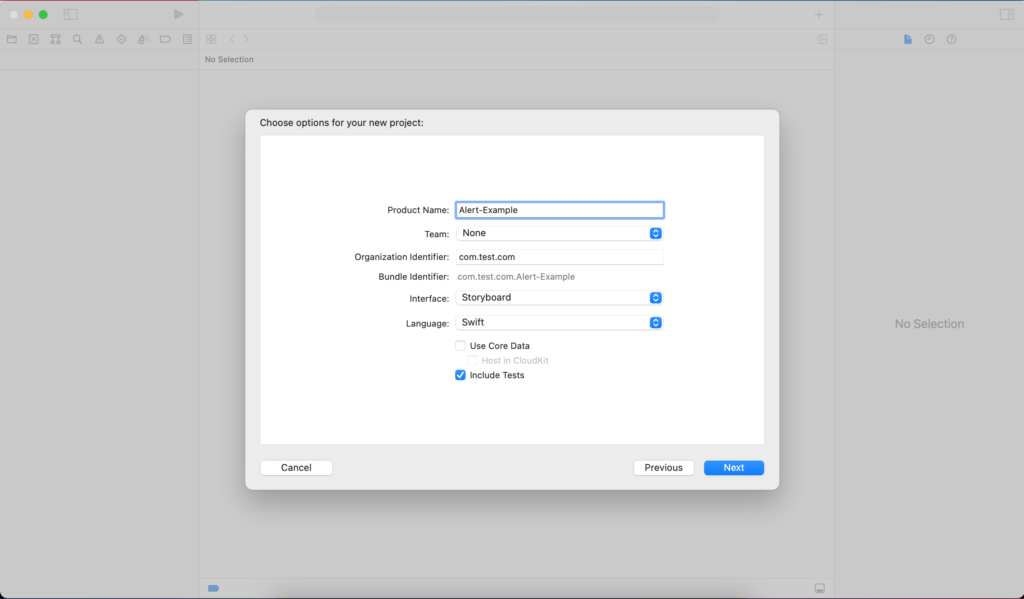
Click on Next button, and open the Main.storyboard, add a button can set the constraints to center of the screen as shown in below image, also change the title of the button to Show Alert.
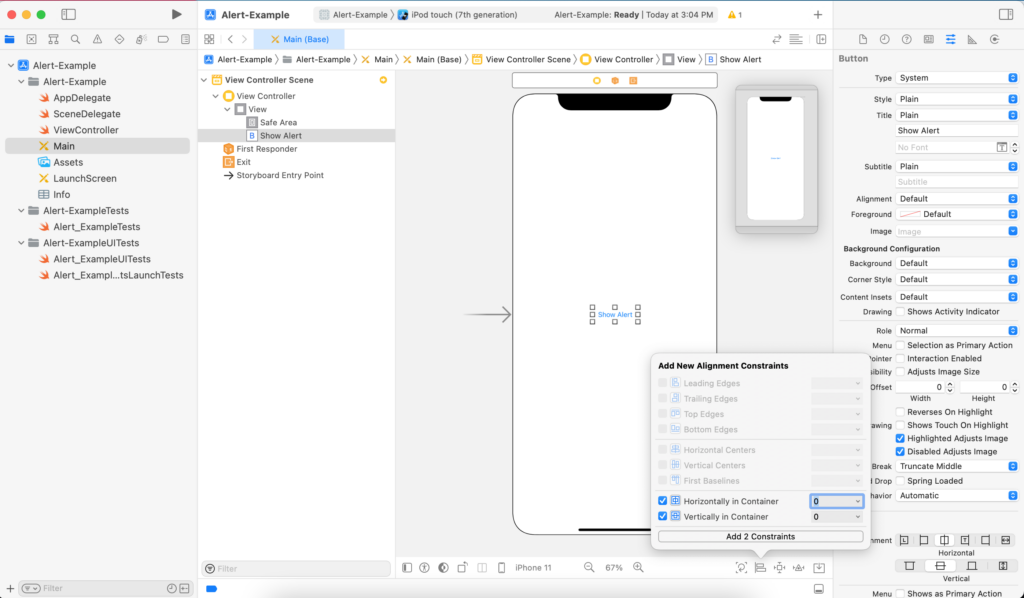
Now add action for the button name it as alertButtonAction(:) one we have configured the basic set up now its time to code.
Now open ViewController.swift class and add the below code.
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
//Button Action
@IBAction func alertButtonAction(_ sender: Any) {
//1.Initilize alert controller
let alertController = UIAlertController(title: "Test Title", message: "Test Message", preferredStyle: .alert)
//2. Set up the actions for alert, below we have configured for ok and cancel actions
let okAction = UIAlertAction(title: "Ok", style: .default) { okAction in
//Call back called when we tap on Ok button on alert
print("Ok Action")
//To dismiss the alert
alertController.dismiss(animated: true, completion: nil)
}
let cancelAction = UIAlertAction(title: "Cancel", style: .destructive) { cancelAction in
//Call back called when we tap on Cancel button on alert
print("Cancel Action")
//To dismiss the alert
alertController.dismiss(animated: true, completion: nil)
}
//3.add the actions to alert controller
alertController.addAction(okAction)
alertController.addAction(cancelAction)
//4. Finally show the alert controller by presening the alert controller
self.present(alertController, animated: true, completion: nil)
}
}
Now lets see how we are configuring the alert view, in iOS alert view will be created using the instance of UIAlertController class that is provided in UIKit, this class is already built and given for developers to use it. All we will do is configure this UIAlertController and present it thats all.
- Here we are initializing the UIAlertController, this class can be initialized with “title” and the “message” texts, this texts will be shown on alert view and also preferred style type of the alert to be shown, basically this is an enum, there are two types, “alert” and “actionSheet“, you can try with both the types, for now you can set it as alert.
- Here we are adding the alert action by creating the instance of UIAlertAction, this is the class provided by UIKit and we just need to configure the title, style for the type of the action, this is an enum we can set any of the type based on the requirement “default“, “cancel” and “destructive“, alert action styles you can set any of the styles for now set default for “okAction” and destructive for “cancelAction”, finally action of the UIAlertAction. Any action on the alert view, let’s say “Ok” button tap or “Cancel” button tap, respective closures callback will get called there will decide what to do based on the user selection.
- Add the instance of the UIAlertAction to alert controller by using the method addAction(:)
- Finally show the alert controller by presenting it by the method present(_ viewControllerToPresent: UIViewController, animated flag: Bool, completion: (() -> Void)? = nil)
Now if you run the app and click on “Show Alert” button.
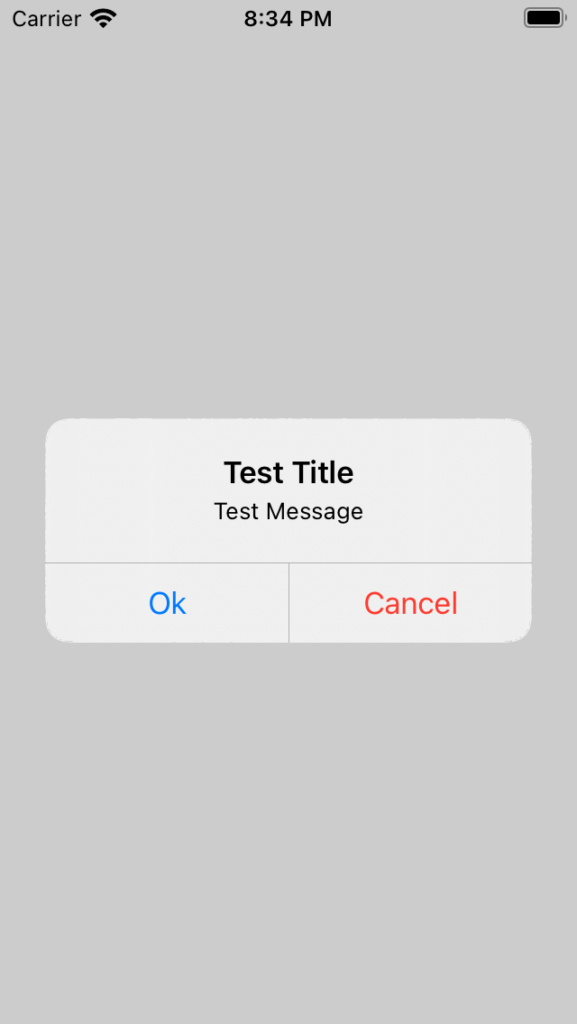